VHS post process Shader (Unity – HLSL) (Late 2022)
fixed4 frag (v2f i) : SV_Target
{
float2 uv = i.uv;
fixed4 srcCol = tex2D(_MainTex, i.uv);
// SCAN LINES SCREEN ------------
float lineWidth = 450;
float4 vColor = float4(0.0f, 0.0f, 0.0f, 1.0f);
vColor += sin(lineWidth * uv.y + frac(_Time.y) * 50) * _scanIntensity;
float4 vColor2 = float4(0.0f, 0.0f, 0.0f, 1.0f);
vColor2 += sin(lineWidth * uv.y + (frac(_Time.y) + 0.05 ) * 50) * _scanIntensity;
vColor = saturate(vColor);
vColor2 = saturate(vColor2);
vColor *= _ColA;
vColor2 *= _ColB;
vColor += vColor2;
// // VIGNETTE ----------------------
float radius = 0.8f;
float circle = 1 - smoothstep(0.0f, radius, length(uv - 0.5f));
vColor -= (1 - circle) * _vignette;
// // GRAIN -------------------------
vColor += random2d(uv * _Time.y) * _noiseIntensity;
// // HORIZONTAL DISTORTION ---------
float p = 0.5; // period
float a = 1.0; // amp
float wave = (a/p) * (p - abs(((uv.y + frac(_Time.x * 2.0f)) % (2*p) - p))); // https://stackoverflow.com/questions/1073606/is-there-a-one-line-function-that-generates-a-triangle-wave
wave = smoothstep(0.98, 0.99, wave);
float2 uvWarp = float2(random1d(uv.y) * wave * 0.05, 0.0f) * pow(circle, 5.0f);
float4 vDistortedFrame = tex2D(_MainTex, uv - uvWarp * 5 * _warpIntensity);
return vDistortedFrame + vColor;
}
Dithering post process Shader (Unity – HLSL) (Late 2022)
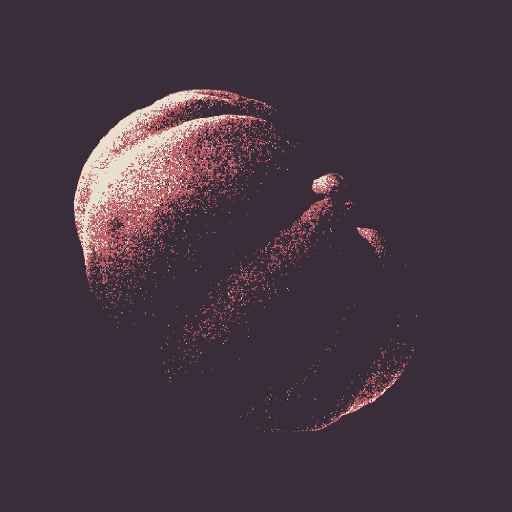
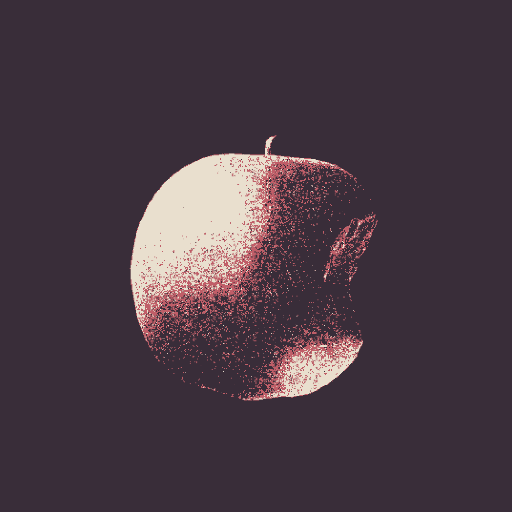
fixed4 frag (v2f i) : SV_Target
{
float aspectRatio = (_ScreenParams.x / _ScreenParams.y);
float invAspectRatio = (_ScreenParams.y / _ScreenParams.x);
float2 Downscale = float2(_Downscale, _Downscale) * float2(aspectRatio, invAspectRatio);
// Screen Downscaling
float2 uv = i.uv;
uv.xy *= Downscale;
uv.xy = floor(uv.xy);
uv.xy /= Downscale;
// Calculate screen grayscale value
float4 screenTex = _MainTex.Sample(point_clamp_sampler, uv);
float lum = dot(screenTex.rgb, float3(0.3, 0.59, 0.11));
lum = smoothstep(_TresholdLow, _TresholdHigh, lum);
// Sample Blue Noise and compare with screen gray value
float2 ditherCoords = uv * _MainTex_TexelSize.xy * _DitherTex_TexelSize.zw * float2(aspectRatio, invAspectRatio);
float ditherLum = _DitherTex.Sample(point_repeat_sampler, ditherCoords * _DitherScale).r;
float ramp = (lum <= clamp(ditherLum, 0.25f, 1.0f)) ? 0.0f : 1.0f;
float3 col = _ColorRamp.Sample(point_clamp_sampler, float2(saturate(ramp), 0.5f));
return float4(col, 1.0f);
}
Metaballs (Unity – HLSL) (Mid 2021)
Shader study of signed distance fields
float sdSphere(float2 p)
{
float2 spheres[2];
spheres[0] = _sphere1;
spheres[1] = _sphere2;
float value = 0;
for(int i = 0; i<2; i++)
{
value += _radius / sqrt(pow((p.x-spheres[i].x), 2) + pow((p.y-spheres[i].y), 2));
}
return value;
}
fixed4 frag (v2f i) : SV_Target
{
// sphere movement
_sphere1.y += 0.5f*sin(_Time.y);
_sphere2.y += 0.5f*sin(_Time.y + 5);
float sd = sdSphere(i.uv);
if(_enableTresh)
{
float stepSd = step(_treshold, sd);
float smooth = smoothstep(0, .2, sd);
float4 lerped = lerp(_ColA, _ColB, smooth);
fixed4 col = lerped * stepSd;
return col;
}
else
{
fixed4 col = float4(sd, sd, sd, 1.0f);
return col;
}
}
Shader Recreation: Apex Legends Gibraltar’s passive. (Mid 2022)
Recreation of Gibraltar’s passive skill in Unreal Engine 5. Textures made in Substance Designer, shader made via material editor nodes inside UE5.
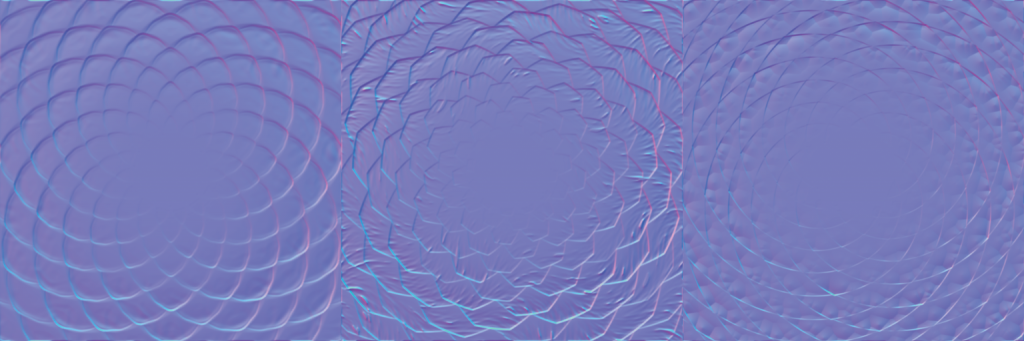
Quantum League: See Through post process material (Late 2020)
Post process material developed inside Unreal Engine 4 for the game Quantum League of Nimble Giant Entertainment.